By using XSL we can write any mapping to a XML, and we can get any type of conversion by using the javax.xml.transform library to do the requried conversion.
Following java source code helps to do the conversion of XML + XSL to any required type.
public void transform(String xslsource, String profileName, String outputFileName, String xmlsource, String profileXMLPath) {//output file name,input xsl,xml source
try {
String fileName = tomcathome + "/webapps/webclient/WEB-INF/jsp/profiles/" + profileName + "/" + outputFileName;
//output file name. This can be any file name
FileOutputStream fileStream = new FileOutputStream(fileName);
//defining the output steam
InputStream in = this.getClass().getResourceAsStream("/lif/webclient/controller/extract/" + xslsource);
//give the xsl source as an input steam
InputStreamReader xsltStream = new InputStreamReader(in);
Source xsltSource = new StreamSource(xsltStream);
TransformerFactory transformerFactory = TransformerFactory.newInstance();
Transformer transformer = transformerFactory.newTransformer(xsltSource);
//cdxml.xml --> dom tree.
FileInputStream xmlStream = new FileInputStream(profileXMLPath + "/" + xmlsource);
DocumentBuilder documentBuilder = DocumentBuilderFactory.newInstance().newDocumentBuilder();
Document doc = documentBuilder.parse(xmlStream);
Source xmlSource = new DOMSource(doc);
Result result = new StreamResult(fileStream);
transformer.transform(xmlSource, result);
fileStream.flush();
fileStream.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace(); //To change body of catch statement use File | Settings | File Templates.
} catch (TransformerConfigurationException e) {
e.printStackTrace(); //To change body of catch statement use File | Settings | File Templates.
} catch (ParserConfigurationException e) {
throw new RuntimeException(e);
} catch (TransformerException e) {
e.printStackTrace(); //To change body of catch statement use File | Settings | File Templates.
} catch (SAXException e) {
e.printStackTrace(); //To change body of catch statement use File | Settings | File Templates.
}
}
In above code you need to provide XML file and the mapping XSL file as an InputStream and after supplying both of these steams to transform method in the instance of the TransformFactory and the required output can be gained from the SteamResult. In the SteamResult constructor you can give the required output as an OutputSteam.
Thursday, March 6, 2008
Remote debugging Apache Tomcat in Fedora with Eclipse
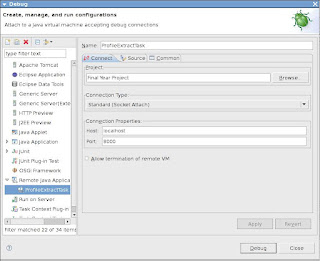
To remote debug a Eclipse project which has deployed in Apache tomcat we need to do following steps.
1. Set the Environment Variables for remote debugging in .bash_profile of Fedora
2. Run Apache tomcat in Remote debugging mode
3. Run Eclipse in Remote debugging mode
So lets look at how we are going to do above steps.
Step 1: Setting up the Environment Varibles for remote debugging in .bash_profile of Fedora
JPDA_ADDRESS=8000
JPDA_TRANSPORT=dt_socket
Step 2: Run Apache Tomcat in Remote debugging mode
To run apache tomcat in remote debugging mode you need to type following additional commands at the end of the starting command
sh $tomcat_home/bin/catalina.sh jpda run
Then we can see that it is listening to the port you have configured in the .bash_profile, Following shows the port which i have configured to listen on tomcat.
Listening for transport dt_socket at address: 8000
Step 3: Run Eclipse in Remote debugging mode
To debug remote debugging in eclipse you need to follow following steps.
1. run -> open debug dialog -> Remote Java Application
2. Add the project which you are going to debug. This project needs to be deployed in the tomcat and the source codes should be exactly same otherwise debugging will do miscellaneous things like debugging comment, space etc
3. Then set the connection properties. The port should be same as the above ports
4. Then press start debugging. Before starting the project you need to start Tomcat.
5. Start the tomcat deployed web application until you reach the debugging point you have declared in the Eclipse
6. When you reach that point then it will ask Eclipse to switch to debugging view.
Friday, October 19, 2007
This Uncomman stuff is cool
i have to face with really unusual stuff with programming with our job interviews. Following shows some examples:
1. Writing hello world without semicolon in C:
#include
int main(){
if(printf("Hello World\n"){
}
}
2. Printing 1 to 100 without using if,for, while or any other loops:
class PrintingWithoutLoopsIf {
public static void main(String args[]){
int z=foo(1);
}
public static int foo(int x) {
System.out.println(x);
x++;
int z=0;
z=(x<101)? foo(x):0;
return 1;
}
}
1. Writing hello world without semicolon in C:
#include
int main(){
if(printf("Hello World\n"){
}
}
2. Printing 1 to 100 without using if,for, while or any other loops:
class PrintingWithoutLoopsIf {
public static void main(String args[]){
int z=foo(1);
}
public static int foo(int x) {
System.out.println(x);
x++;
int z=0;
z=(x<101)? foo(x):0;
return 1;
}
}
Thursday, October 11, 2007
About Final Year project

We started our final year project implementation. We were able to get the locations point out in our
Google maps. It was surprising to us because when we started implementation without a kick it's very
lazy. Now we are developing very quickly and we are interested about it very much rather than earlier time. this is some example pick which we have already build.
Subscribe to:
Posts (Atom)